How to Configure localhost HTTPS for your Vue App
Problem Statement
How do I use the Vue development server to serve requests from https while working on localhost?
As apps mature and add functionality such as authentication or integrations with other products, engineers will inevitable need to run code locally using https. While configuring https for localhost development is OS dependent due to the inherent nature of certificate management, this post will focus on configuring a Vue App built using the Vue CLI on Windows.
Before going forward, I want to make sure to give credit to Ruben Vermeulen for his post on Medium and his generate trusted ssl certificate repo on GitHub. I’ll build off a lot of his work in an effort to streamline the configuration on a Windows machine utilizing Chocolatey to install OpenSSL and running a powershell command within an Vue CLI project to generate the ssl cert.
In order to run Vue 3 over SSL we will need to
- Generate and configure an SSL certificate
- Install the certificate to a local Certificate Authority (CA), and
- Configure the Vue dev sever to use our use our generated certificate.
Taken from the perspective of a Vue app created using the CLI, we will tell Vue to use a specific cert that we generated locally, and that cert will tell the browser to look to the local machine as the Certificate Authority for cert verification.
You can find all of the code for this project on my GitHub at https://github.com/BrianMikinski/vuessl
Plan of Action
- Clone vuessl Vue Starter Project from my GitHub
- Install Chocolatey
- Install OpenSSL
- OpenSSL Certificate Creation
- Certificate Installation
- Update npm run-script
Clone vuessl Starter Project from GitHub
Rather than start from scratch, I already have an Vue project configured to fast track building your local https cert and configure npm to start the Vue development server in ssl mode. Clone this project from GitHub using
1
git clone https://github.com/BrianMikinski/vuessl.git
Now, let’s get started building our cert and configuring our Vue App!
Install Chocolatey
Prior to creating an SSL cert to be installed in your local Certificate Authority, we need to first install OpenSSL.
Running on Windows, you can install OpenSSL using a fantastic package manager for Windows called Chocolatey. Go and grab the following PowerShell script that can be found at Installing Chocolatey and run them under an admin PowerShell window. After installing Chocolatey as a package manager, we’re now ready to install OpenSSL (Note: you definitely will need to close your PowerShell window and may even need to restart your computer after installing Chocolatey to get the $PATH environment variable to properly pick up)
1
Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://community.chocolatey.org/install.ps1'))
Install OpenSSL
With Chocolatey installed, we’re ready to install OpenSSL (using Chocolatey) which will allow us to generate certificates and keys for the Vue CLI to us.
1
choco install openssl
To test that open ssl was properly installed, run the following command
1
openssl version
If you recieved output stating the version of OpenSSL that you are running, you should be good to go.
OpenSSL Certificate Creation
With OpenSSL installed the next step is to build a certificate for local installation to the correct certificate authority. To generate the local cert, navigate to the vuessl repo folder that was cloned from github and run the generateSslCert.ps1 PowerShell script. This script will call OpenSSL passing in cert definition defined in ./ssl/certdef.cnf and place the certificate along with a key in the ./ssl folder of the ngssl starter project.
certdef.cnf
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
[req]
default_bits = 2048
prompt = no
default_md = sha256
x509_extensions = v3_req
distinguished_name = dn
[dn]
C = US
ST = TX
L = HTX
O = IT
OU = IT Department
emailAddress = webmaster@ustxhtx.com
CN = localhost
[v3_req]
subjectAltName = @alt_names
[alt_names]
DNS.1 = *.localhost
DNS.2 = localhost
Generate SSL Cert PowerShell Script
1
./generateSslCert.ps1
With the certificate and key created, we can now move on to installing the cert and updating our packages.json script
Certificate Installation
To install the certificate on Windows, navigate to the vuessl/ssl/ folder and double click on the certificate. This will open up the Certificate Import Wizard. On the Certificate Import Wizard, click through the wizard to place the certificate in the following location
Install Certificate > Current User > Place all Certificates in the following store > Browse > Trusted Root Certification Authorities > Ok
1. Click Install Certificate
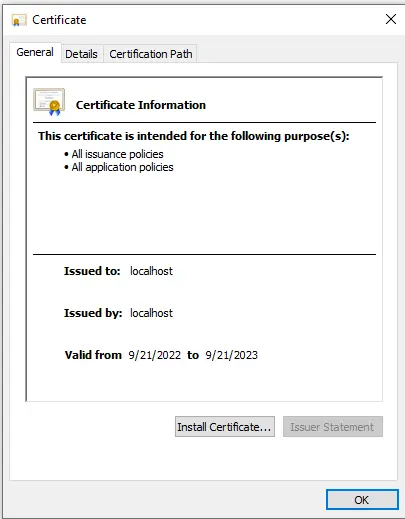
2. Select Current User
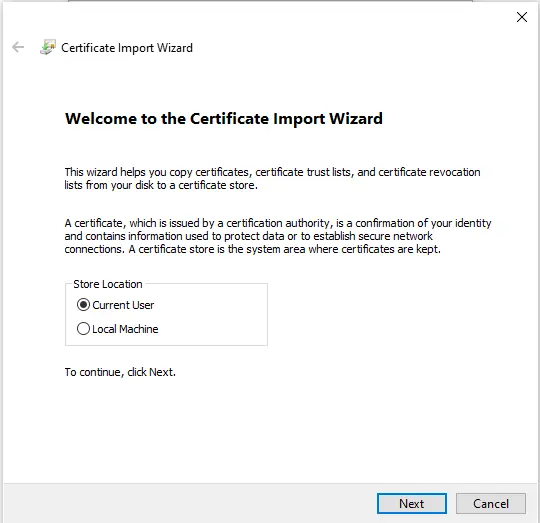
3. Select Place all Certificates in the following store, Browse
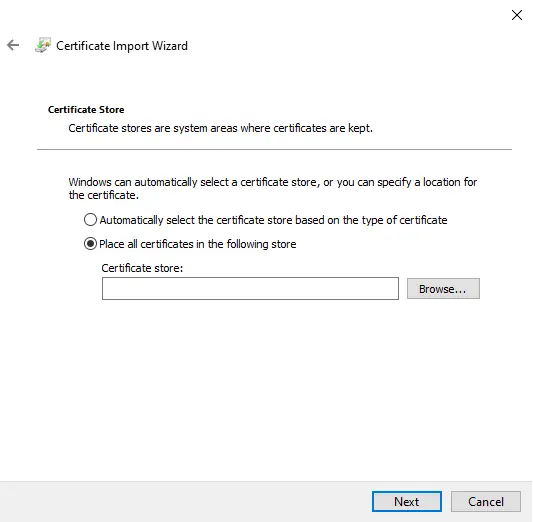
4. Select Trusted Root Certification Authorities
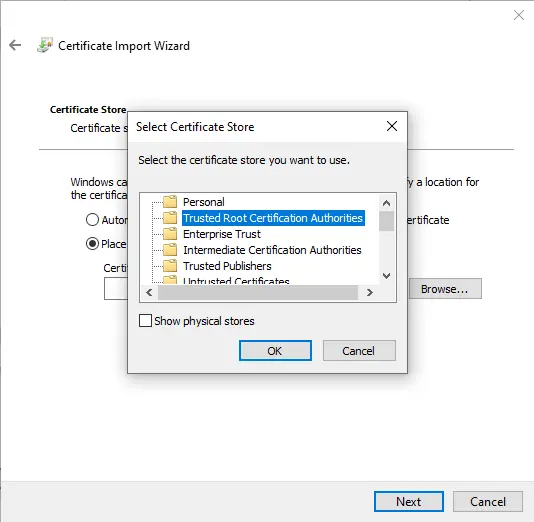
5. Select Finish
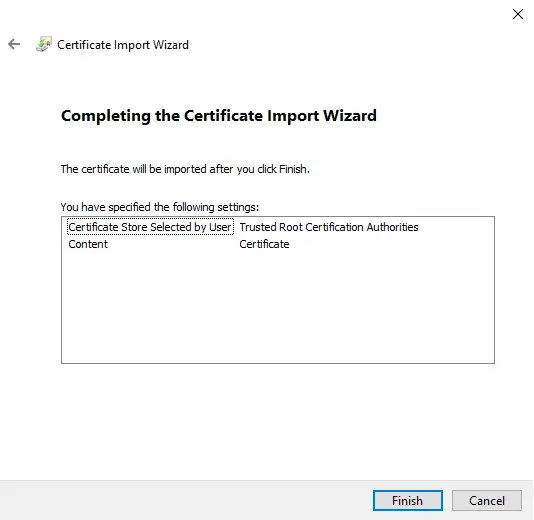
Update the vue.config.js file
Everything is now in place to start running Vue with SSL enabled on localhost. To bring localhost ssl to completion, we need to update the Vue dev server to utilize the generated certificate and key. While we will be editing a config property called devServer
in the vue.config.js
file, Underneath the hood this property maps back to Webpack 5’s aptly named DevServer. Crack open the vue.config.js
file and make the following edits -
packages.json scripts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
const { defineConfig } = require('@vue/cli-service')
// add require for fs node library
const fs = require('fs');
module.exports = defineConfig({
transpileDependencies: true,
// tell the Webpack 5 devServer where to find the server key and cert
devServer: {
https: {
key: fs.readFileSync('./ssl/server.key'),
cert: fs.readFileSync('./ssl/server.crt'),
}
}
})
Finally, with these updates we’re ready to run the app. Start up the app by running
1
npm run serve
If all goes well you should see npm compile your app, start the Webpack5 DevServer and serve your Vue app flawlessly using https!
Feel free to leave a comment below, thanks for learning along with me and happy coding!
Resources
Reuben Vermeulen